PIL
实现效果如下:

from PIL import Image,ImageFont,ImageDraw
import os
from PIL import Image,ImageFont,ImageDraw
import pandas as pd
# text=str(pd.read_csv(r'D:\output.csv',encoding='gbk'))
text = u"这是一段测试文本,test 123。"
im = Image.new("RGB", (300, 50), (255, 255, 255))
dr = ImageDraw.Draw(im)
font = ImageFont.truetype(os.path.join("fonts", "msyh.ttf"), 14)
dr.text((10, 5), text, font=font, fill="#000000")
im.show()
im.save(r'D:\output.png')
pygame
实现效果如下:

#先导入所需的包
import pygame
import os
import pandas as pd
pygame.init() # 初始化
B = """你好
一起郊游呀""" # 变量B需要转图片的文字
text = u"{0}".format(B) # 引号内引用变量使用字符串格式化
# text=str(pd.read_csv(r'D:\output.csv',encoding='gbk'))
print(text)
#设置字体大小及路径
font = pygame.font.Font(os.path.join("C:/Windows/Fonts", "msyh.ttf"), 26)
# font = pygame.font.Font(os.path.join("/Users/akun/Library/Fonts", "msyh.ttf"), 26)
#设置位置及颜色
rtext = font.render(text, True, (0, 0, 0), (255 ,255 ,255))
#保存图片及路径
pygame.image.save(rtext, "D:\output.png")
pygame文字换行
需提前准备背景图片,并存放指定位置:
Image.open(r'D:\a.jpg') # 读取背景图片
实现效果如下:
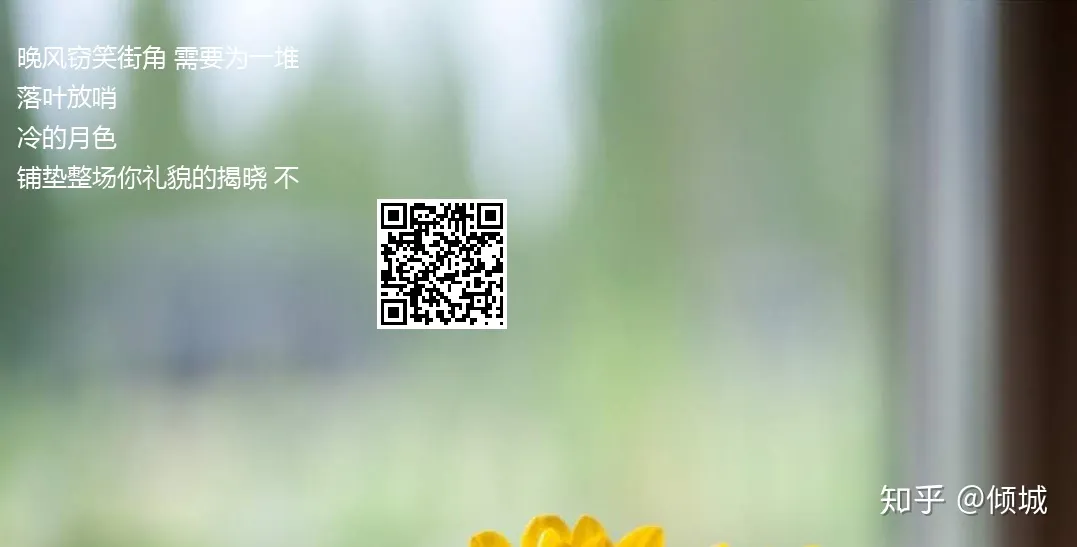
from PIL import Image, ImageDraw, ImageFont
import qrcode
import pygame
backMode = {
# 背景图属性,我的背景图上需要添加一个二维码和多个文本框
# 背景图属性,我的背景图上需要添加一个二维码和多个文本框
"back_url": "D:\\a.jpg",
"size": (550, 363),
"QR": {
# 二维码属性
"frame": (130, 130), # 大小
"position": (380, 200), # 位置
},
"text": [{
# 文本框属性
"size": 25, # 字号
"ttf": "FZXBSJW.TTF", # 字体
"color": "", # 颜色
"position": (20, 40),
"frame": (300, 20),
}, {
"size": 25,
"ttf": "FZXBSJW.TTF",
"color": "",
"position": (20, 80),
"frame": (300, 20),
}, {
"size": 25,
"ttf": "FZXBSJW.TTF",
"color": "",
"position": (20, 120),
"frame": (300, 20),
}, {
"size": 25,
"ttf": "FZXBSJW.TTF",
"color": "",
"position": (20, 160),
"frame": (300, 20),
}, ],
}
def make_QR(content, sizeW=0, sizeH=0): # 创建二维码
qr = qrcode.QRCode(version=3, box_size=3, border=1, error_correction=qrcode.constants.ERROR_CORRECT_H)
qr.add_data(content)
qr.make(fit=True)
img = qr.make_image()
if sizeW == 0 and sizeH == 0:
return img
w, h = img.size
if sizeW < w or sizeH < h:
return None
img = img.resize((sizeW, sizeH), Image.ANTIALIAS)
return img
def com_pic(topimg, backimg, position): # 合并图片
nodeA = position
w, h = topimg.size
nodeB = (position[0] + w, position[1] + h)
backimg.paste(topimg, (nodeA[0], nodeA[1], nodeB[0], nodeB[1]))
return backimg
import os
def write_line(backimg, text, tmode): # 给单个文本框填充数据
# myfont = ImageFont.truetype(tmode["ttf"], size=tmode["size"])
myfont=ImageFont.truetype(os.path.join("fonts", "msyh.ttf"), size=tmode["size"])
draw = ImageDraw.Draw(backimg)
tend = len(text)
while True:
text_size = draw.textsize(text[:tend], font=myfont) # 文本图层的尺寸
# print(text_size)
if text_size[0] <= tmode["frame"][0]:
break
else:
tend -= 1 # 文本太长,调整文本长度
draw.text((tmode["position"][0], tmode["position"][1]), text[:tend], font=myfont)
return backimg, tend
def write_text(img, text, tmodeList): # 写文本
tlist = text.split("\n")
mnum = 0
draw = ImageDraw.Draw(img)
for t in tlist:
tbegin = 0
tend = len(t)
while True:
img, tend = write_line(img, t[tbegin:tend], tmodeList[mnum])
mnum += 1
if tbegin + tend == len(t) or mnum == len(tmodeList):
break
else:
tbegin = tbegin + tend
tend = len(t)
if mnum == len(tmodeList):
break
return img
def make_pic(mode, text, url):
img = Image.open(mode["back_url"])#读取背景图片
img = Image.open(r'D:\a.jpg') # 读取背景图片
QR_res = make_QR(url, mode["QR"]["frame"][0], mode["QR"]["frame"][1]) # 创建二维码
img = com_pic(QR_res, img, mode["QR"]["position"]) # 合成1
img = write_text(img, text, mode["text"]) # 写文本
img.show()
img.save(r'D:\output.png', quality=100)
if __name__=='__main__':
make_pic(backMode, "晚风窃笑街角 需要为一堆落叶放哨\n冷的月色\n铺垫整场你礼貌的揭晓 不爱我就拉倒", "http://bd.kuwo.cn/yinyue/41230185")
exit()
转载于:https://zhuanlan.zhihu.com/p/149445150